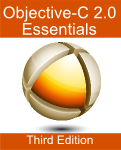
The following issues are known to exist in this edition of the book:
Chapter 4 - Objective-C Data Types
The binary representation of 99 is incorrectly listed as 1010110011. This should be 1100011.Section 5.3 - Type Casting Objective-C Variables
The text in this section incorrectly states that type casting will round the result to the nearest whole number:double balance = 100.54; double interestRate = 5.78; double result; result = (int) balance * (int) interestRate; NSLog(@"The result is %f", result);The above code actually truncates the numbers rather than rounding them (thereby multiplying 100 * 5). The correct code to perform rounding is as follows:
double balance = 100.54; double interestRate = 5.78; double result; result = round(balance) * round(interestRate); NSLog(@"The result is %f", result);
Section 13.4 - Declaring an Objective-C Class Interface
The following steps were omitted from section 13.4 immediately after Figure 13-1:"Since we are adding a new class to the project, select the Objective-C class entry before clicking Next. On the subsequent panel, enter BankAccount into the Class field and change the Subclass of menu to NSObject. Click Next, select a location for the new class files and click on Create."
Section 16.5 - Overriding Inherited Methods
The final code example for the main.m file incorrectly imports "SavingsAccount" instead of "SavingsAccount.h". This code example should read:#importIf you have encountered a problem or error in the book that is not listed above, please contact us as at feedback@ebookfrenzy.com and we will work to resolve the issue for you as quickly as possible.#import "BankAccount.h" #import "SavingsAccount.h" int main(int argc, const char * argv[]) { @autoreleasepool { BankAccount *account1; SavingsAccount *account1 = [[SavingsAccount alloc] init]; [account1 setAccount: 4543455 andBalance: 3010.10]; [account1 setInterestRate: 0.001]; float interestEarned = [account1 calculateInterest]; NSLog (@"Interest earned = %f", interestEarned); [account1 displayAccountInfo]; } return 0; }